Linux “Expect” Command – Complete Guide
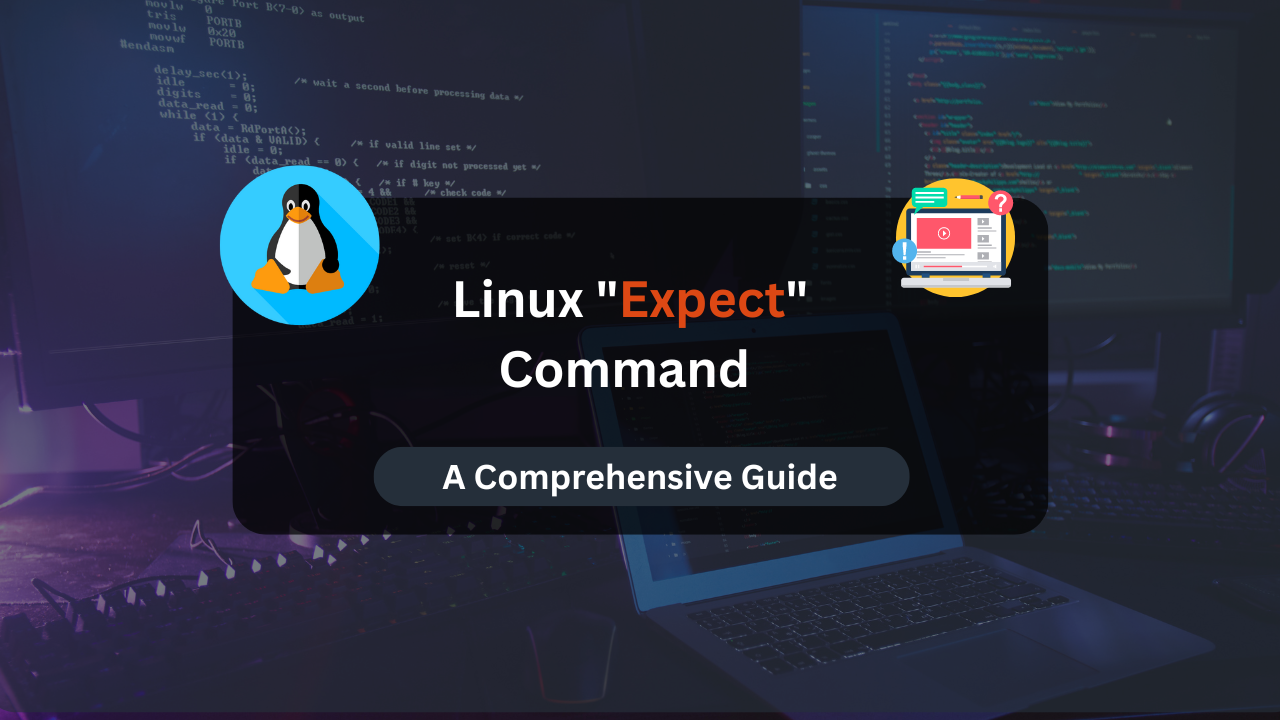
The Expect command in Linux is a powerful tool used to automate interactions with programs that require user input. It’s particularly useful for scripting interactive applications, automating repetitive tasks, and testing user interfaces.
In this guide, we’ll delve into the intricacies of the Expect command, provide practical examples, and explore advanced usage scenarios to help you harness its full potential.
What is Expect?
Expect is a program that “talks” to other interactive programs according to a script. Following the script, Expect knows what can be expected from a program and what the correct response should be. It was designed to automate control of interactive applications such as telnet, ftp, passwd, fsck, rlogin, and others.
Key Features of Expect
- Automate Interactive Applications: Automate tasks that require user interaction.
- Test Scripts: Create scripts to test interactive applications.
- Flexible Control: Offers precise control over the input/output of applications.
Installing Expect
Expect is available in the package repositories of most Linux distributions. To install it, use the following commands based on your distribution:
On Debian-based Systems (e.g., Ubuntu)
sudo apt-get update
sudo apt-get install expect
On Red Hat-based Systems (e.g., CentOS, Fedora)
sudo yum install expect
On Arch Linux
sudo pacman -S expect
Verifying the Installation
To verify that Expect is installed correctly, you can check its version:
expect -v
This command should display the installed version of Expect.
Basic Usage of Expect
Expect scripts are written in Tcl (Tool Command Language), which is the scripting language Expect is built upon. The basic structure of an Expect script involves sending commands to a program and waiting for specific responses.
A Simple Expect Script
Here’s a simple Expect script to automate logging into a remote server via SSH:
#!/usr/bin/expect
set timeout 20
set user "username"
set password "password"
set host "hostname"
spawn ssh $user@$host
expect "password:"
send "$password\r"
expect "$ "
send "ls -l\r"
expect "$ "
send "exit\r"
Explanation
#!/usr/bin/expect
: Specifies the script interpreter.set timeout 20
: Sets the timeout for the script.set user "username"
: Sets the username variable.set password "password"
: Sets the password variable.set host "hostname"
: Sets the hostname variable.spawn ssh $user@$host
: Initiates the SSH connection.expect "password:"
: Waits for the password prompt.send "$password\r"
: Sends the password.expect "$ "
: Waits for the shell prompt.send "ls -l\r"
: Sends thels -l
command.expect "$ "
: Waits for the shell prompt.send "exit\r"
: Exits the SSH session.
Running the Script
To run the Expect script, save it to a file (e.g., ssh_login.exp
) and make it executable:
chmod +x ssh_login.exp
./ssh_login.exp
Advanced Expect Commands
Interacting with Multiple Prompts
Expect can handle multiple prompts and responses in a single script. Here’s an example of an Expect script that handles both username and password prompts:
#!/usr/bin/expect
set timeout 20
set user "username"
set password "password"
set host "hostname"
spawn ssh $host
expect {
"username:" {
send "$user\r"
exp_continue
}
"password:" {
send "$password\r"
}
}
expect "$ "
send "ls -l\r"
expect "$ "
send "exit\r"
Using Variables and Control Structures
Expect scripts can use variables and control structures (loops, conditionals) to make the scripts more dynamic and flexible. Here’s an example that demonstrates the use of a loop to automate multiple SSH logins:
#!/usr/bin/expect
set timeout 20
set hosts {"host1" "host2" "host3"}
set user "username"
set password "password"
foreach host $hosts {
spawn ssh $user@$host
expect "password:"
send "$password\r"
expect "$ "
send "uptime\r"
expect "$ "
send "exit\r"
}
Logging Output
Expect provides logging capabilities to capture the output of the interactions. This can be useful for debugging and record-keeping. Here’s an example:
#!/usr/bin/expect
set timeout 20
set user "username"
set password "password"
set host "hostname"
log_file -noappend /path/to/logfile.log
spawn ssh $user@$host
expect "password:"
send "$password\r"
expect "$ "
send "ls -l\r"
expect "$ "
send "exit\r"
Affordable VPS Hosting With Dracula Servers
Looking for reliable and budget-friendly Virtual Private Server (VPS) hosting? Look no further than Dracula Servers. Dracula Servers offers a range of VPS hosting plans tailored to meet diverse needs. With competitive pricing, robust performance, and a user-friendly interface, it’s an excellent choice for individuals and businesses alike.
Explore the Dracula Servers website to discover hosting solutions that align with your requirements and take your online presence to new heights with their affordable and efficient VPS hosting services.
Visit Dracula Servers and experience reliable VPS hosting without breaking the bank.
Real-World Applications
Automating FTP Sessions
Expect can automate FTP sessions by interacting with the FTP client. Here’s an example script to upload a file to an FTP server:
#!/usr/bin/expect
set timeout 20
set user "ftpuser"
set password "ftppass"
set host "ftphost"
set file "/path/to/file"
spawn ftp $host
expect "Name*:"
send "$user\r"
expect "Password:"
send "$password\r"
expect "ftp>"
send "put $file\r"
expect "ftp>"
send "bye\r"
Automating Software Installation
Automating software installations that require user input during the process can save time and ensure consistency. Here’s an example of automating a software installation:
#!/usr/bin/expect
set timeout 60
spawn sudo apt-get install somepackage
expect "Do you want to continue? [Y/n]"
send "Y\r"
expect eof
Automated Testing
Expect is widely used for automated testing of interactive applications. Here’s a simple example of testing a CLI application:
#!/usr/bin/expect
set timeout 20
spawn ./mycliapp
expect "Enter your name:"
send "John Doe\r"
expect "Enter your age:"
send "30\r"
expect "Welcome, John Doe"
send "exit\r"
Best Practices for Writing Expect Scripts
Handle Errors Gracefully
Ensure your scripts can handle errors and unexpected situations gracefully. Use timeout and error handling mechanisms to manage such scenarios.
#!/usr/bin/expect
set timeout 20
set user "username"
set password "password"
set host "hostname"
spawn ssh $user@$host
expect {
"password:" {
send "$password\r"
}
timeout {
puts "Connection timed out"
exit 1
}
eof {
puts "Connection failed"
exit 1
}
}
expect "$ "
send "ls -l\r"
expect "$ "
send "exit\r"
Modularize Your Scripts
Break down complex scripts into smaller, reusable procedures. This improves readability and maintainability.
#!/usr/bin/expect
proc login {user password host} {
spawn ssh $user@$host
expect "password:"
send "$password\r"
expect "$ "
}
proc run_command {command} {
send "$command\r"
expect "$ "
}
# Main script
set user "username"
set password "password"
set host "hostname"
login $user $password $host
run_command "ls -l"
run_command "uptime"
send "exit\r"
Conclusion
The Expect command in Linux is a versatile and powerful tool for automating interactions with programs that require user input. By mastering Expect, you can automate repetitive tasks, streamline workflows, and enhance productivity. This guide has covered the basics of Expect, advanced usage scenarios, real-world applications, and best practices. With these insights, you are well-equipped to leverage Expect for your automation needs. Practice creating and running Expect scripts, explore its vast capabilities, and integrate it into your daily Linux administration tasks to see its full potential in action.
Check out More Linux Tutorials Here!
Subscribe
Login
0 Comments
Oldest