How to Set and Use Environment Variables in Bash Script
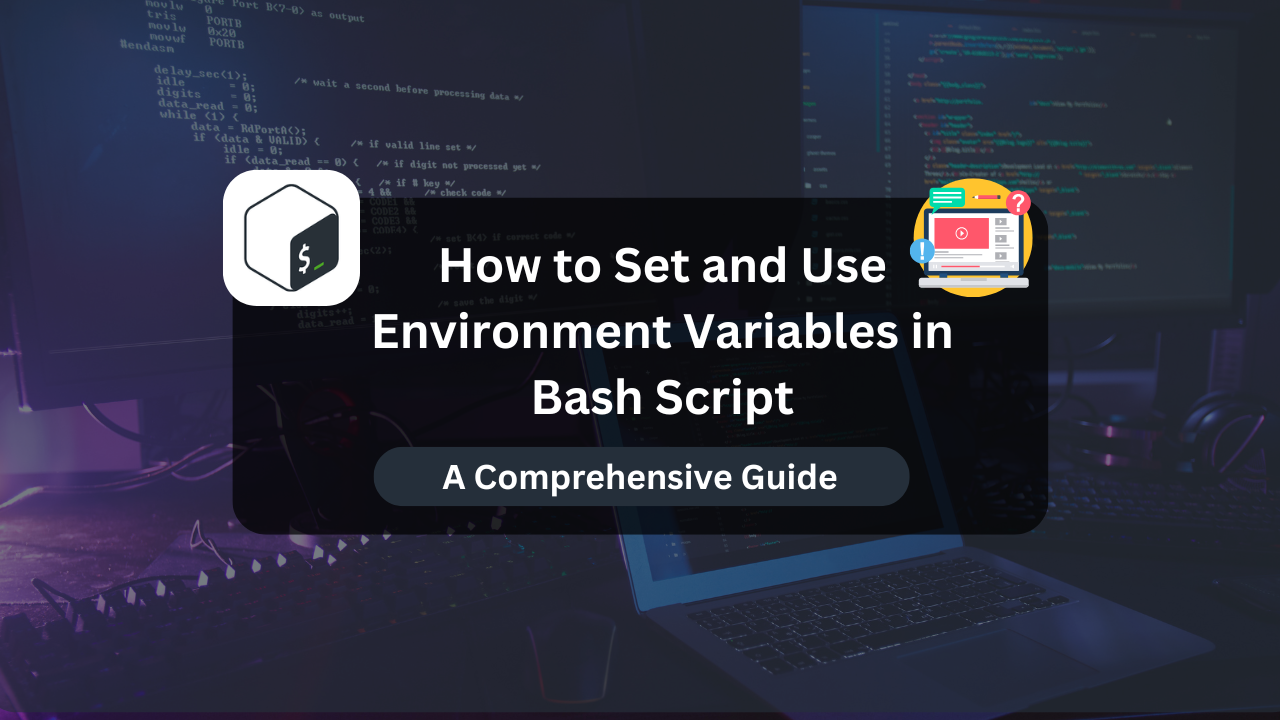
Environment variables are essential components in a Linux system. They allow you to store values that can be referenced and used by applications, scripts, and the operating system itself. When working with Bash scripts, environment variables play a crucial role in making your scripts flexible, adaptable, and efficient. This guide will cover how to set and use environment variables in Bash scripts, complete with examples, best practices, and troubleshooting tips.
Understanding Environment Variables
Environment variables are key-value pairs that store data used by applications and processes running in a Linux environment. They are typically set in the shell or system and can be accessed by programs, scripts, and other shell commands.
Common examples include:
PATH
: Stores the directories where the shell looks for executable files.HOME
: Represents the current user’s home directory.USER
: The username of the current user.
Why Use Environment Variables in Bash Scripts?
Using environment variables in Bash scripts has several benefits:
- Flexibility: Variables can make your script adaptable to different environments.
- Reuse: Environment variables allow you to reference values without hard-coding them.
- Security: Sensitive information, like API keys or passwords, can be stored in environment variables.
Setting Environment Variables in Bash
Method 1: Setting Variables Temporarily
A temporarily set environment variable is only available within the current shell session. To set a temporary environment variable, use the following syntax:
export VARIABLE_NAME="value"
For example:
export GREETING="Hello, World!"
To view the variable’s value, use the echo
command:
echo $GREETING
Method 2: Setting Variables in a Script
To use environment variables in a Bash script, you can define them within the script itself. Here’s an example of defining and using an environment variable within a script:
#!/bin/bash
export GREETING="Hello from the script!"
echo $GREETING
When you run this script, it will output the greeting message. Note that the export
command makes the variable available to other scripts or commands run from within this script.
Method 3: Setting Persistent Environment Variables
To make an environment variable persistent across all sessions, you need to add it to your shell’s configuration file (e.g., ~/.bashrc
or ~/.bash_profile
for Bash users).
- Open your shell’s configuration file:
nano ~/.bashrc
- Add the export command with your variable:
export GREETING="Hello, World!"
- Save the file and exit the editor.
- Apply the changes:
source ~/.bashrc
This variable will now be available in all new shell sessions.
Using Environment Variables in Bash Scripts
Once you have set your environment variables, you can use them in your scripts by referencing them with the $
symbol.
Example 1: Simple Variable Usage
Let’s create a script that uses the USER
and HOME
environment variables to print a welcome message:
#!/bin/bash
echo "Hello, $USER!"
echo "Your home directory is $HOME."
Example 2: Using Variables to Store Paths
Environment variables are handy for defining paths. Here’s an example script that uses environment variables to set and use custom directory paths:
#!/bin/bash
export BACKUP_DIR="/backup"
export LOG_DIR="/var/log/myapp"
echo "Backing up files to $BACKUP_DIR..."
echo "Logs are saved in $LOG_DIR"
Example 3: Passing Arguments as Environment Variables
You can pass values to a script at runtime and store them in environment variables. Here’s a script that takes an argument and uses it as an environment variable:
#!/bin/bash
export FILE_NAME=$1
echo "Processing file: $FILE_NAME"
To run this script and pass an argument:
./script.sh example.txt
The output will display:
Processing file: example.txt
Exporting Variables to Sub-Shells
When you define an environment variable in a script, it is only available within that script by default. If you want to make a variable available to sub-shells or other scripts executed from the main script, you need to use the export
command.
Example
In this example, VARIABLE
is available within sub_script.sh
because of the export
command:
#!/bin/bash
export VARIABLE="shared_value"
./sub_script.sh
Within sub_script.sh
:
#!/bin/bash
echo "The value of VARIABLE is: $VARIABLE"
Best Practices for Using Environment Variables
1. Use Uppercase Names
By convention, environment variables are written in uppercase letters. This makes them easy to identify and differentiate from regular variables.
2. Avoid Overwriting System Variables
Be cautious not to overwrite system variables (like PATH
, HOME
, or USER
) unless you have a specific purpose and understand the consequences. Overwriting critical variables can lead to unexpected behavior.
3. Use Meaningful Names
Using descriptive names for your environment variables helps improve readability and maintainability. For example, instead of VAR1
, use something like BACKUP_DIR
.
4. Keep Sensitive Information Secure
For sensitive information like passwords or API keys, consider storing them in a separate, secured environment file (e.g., .env
) and load them into your script as needed.
5. Clean Up Temporary Variables
If a variable is only needed for a specific portion of your script, consider unsetting it when it’s no longer required:
unset VARIABLE_NAME
Loading Environment Variables from a File
Sometimes, you may have a set of environment variables stored in a file that you want to load into your script. This is common for managing configurations or secrets in a project.
Example: Loading Variables from an .env
File
- Create a file named
.env
with the following content:API_KEY="your_api_key_here" DATABASE_URL="your_database_url_here"
- Load the variables into your script using the
source
command:#!/bin/bash source .env echo "API_KEY is $API_KEY" echo "DATABASE_URL is $DATABASE_URL"
This script will output the values of API_KEY
and DATABASE_URL
from the .env
file.
Using Environment Variables in Conditional Statements
Environment variables can be used in conditional statements to control script behavior based on certain conditions.
Example
In this example, the script checks if a variable named DEBUG
is set to “true” before executing a debug-specific command.
#!/bin/bash
export DEBUG=true
if [ "$DEBUG" = "true" ]; then
echo "Debug mode is enabled."
fi
Troubleshooting Environment Variable Issues
When working with environment variables, you may encounter a few common issues. Here’s how to address them:
1. Variable Not Found
If your script cannot find an environment variable, ensure:
- The variable is exported properly if needed by sub-scripts.
- You are referencing it correctly with
$
(e.g.,$VARIABLE_NAME
). - You have reloaded the shell configuration file after making changes (
source ~/.bashrc
).
2. Variable Not Persisting Across Sessions
If your environment variable does not persist across sessions, it’s likely because it’s not defined in a persistent file like .bashrc
or .bash_profile
. Add it to one of these files to make it persistent.
3. Unexpected Output
If a variable’s value contains spaces or special characters, enclose the variable in quotes:
export PATH="/usr/local/bin:/usr/bin"
echo "$PATH"
Conclusion
Environment variables are an invaluable tool in Bash scripting, allowing you to create flexible and adaptable scripts that can work in various environments. By setting, exporting, and using environment variables effectively, you can significantly enhance the functionality and maintainability of your Bash scripts.
This guide covered setting environment variables temporarily and permanently, using them within scripts, best practices, and troubleshooting common issues. Armed with this knowledge, you can start building more dynamic and powerful scripts for your Linux environment.
Subscribe
Login
0 Comments
Oldest