How to Make a Script Executable in Linux Using the `chmod` Command
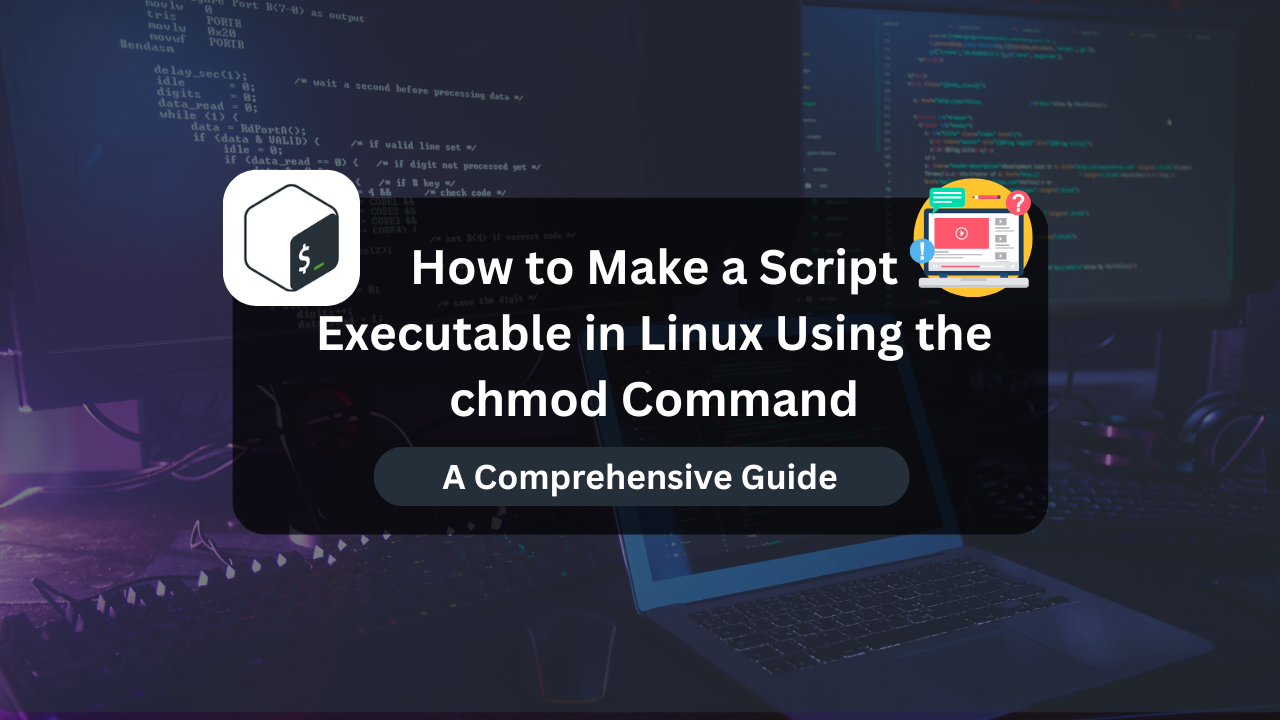
Linux provides a robust and flexible environment for scripting and automation. One of the most essential steps in running a script is making it executable. By default, when you create a script file, it lacks execution permissions, meaning you can’t run it directly. The chmod
command is the tool you use to modify these permissions and make your script executable.
In this article, we’ll dive deep into the concept of file permissions in Linux, explore how to use the chmod
command to make a script executable, and discuss best practices to ensure your scripts are secure and efficient.
Understanding File Permissions in Linux
Before making a script executable, it’s crucial to understand how file permissions work in Linux. File permissions define who can read, write, or execute a file.
File Permission Basics
Each file in Linux has three types of permissions:
- Read (
r
): Allows the file’s contents to be read. - Write (
w
): Allows the file to be modified. - Execute (
x
): Allows the file to be executed as a program.
Permissions are set for three categories of users:
- Owner: The user who owns the file.
- Group: Users in the same group as the file.
- Others: Everyone else.
Viewing File Permissions
To check a file’s permissions, use the ls -l
command:
ls -l script.sh
Output:
-rw-r--r-- 1 user group 1024 Nov 10 10:00 script.sh
Here’s what each section means:
-rw-r--r--
: File permissions.r
andw
for the owner (read and write).r
for the group (read only).r
for others (read only).
1
: Number of links to the file.user
: The owner of the file.group
: The group associated with the file.1024
: File size in bytes.Nov 10 10:00
: Last modification date and time.script.sh
: The file name.
If a script lacks the x
(execute) permission, it can’t be run directly. This is where the chmod
command comes in.
What is the chmod
Command?
The chmod
command, short for change mode, is used to modify the permissions of a file or directory. It allows you to grant or revoke read, write, and execute permissions.
Basic Syntax
chmod [options] mode file
mode
: Specifies the new permissions (e.g.,+x
to add execute permissions).file
: The file or directory whose permissions you want to change.
Modes of Permission Changes
- Symbolic Mode: Uses symbols like
+
,-
, and=
to modify permissions.+
adds a permission.-
removes a permission.=
sets specific permissions.
- Octal Mode: Uses numeric codes to set permissions directly.
Making a Script Executable with chmod
Step 1: Create a Script
Let’s create a simple script as an example:
nano script.sh
Add the following content:
#!/bin/bash
echo "Hello, Linux World!"
Save the file by pressing Ctrl+O
, then Enter
, and exit with Ctrl+X
.
The #!/bin/bash
at the beginning specifies the script’s interpreter, in this case, Bash.
Step 2: Check Script Permissions
Run the following command to view the script’s current permissions:
ls -l script.sh
Output:
-rw-r--r-- 1 user group 44 Nov 10 10:30 script.sh
Notice the absence of the x
(execute) permission.
Step 3: Add Execute Permission
To make the script executable, use the chmod
command:
chmod +x script.sh
Recheck the permissions:
ls -l script.sh
Output:
-rwxr-xr-x 1 user group 44 Nov 10 10:30 script.sh
Now the script has x
permissions for the owner, group, and others.
Running the Executable Script
After making the script executable, you can run it using one of the following methods:
- Direct Path:
./script.sh
- Absolute Path:
/home/user/script.sh
- Add to
$PATH
: Move the script to a directory in your$PATH
(e.g.,/usr/local/bin
) to run it like a regular command:sudo mv script.sh /usr/local/bin/ script.sh
Advanced chmod
Usage for Scripts
Using Octal Mode
Octal numbers provide a direct way to set permissions. Each digit represents a permission set for the owner, group, and others.
4
: Read2
: Write1
: Execute
For example:
chmod 755 script.sh
: Grants full permissions to the owner and read/execute permissions to the group and others.chmod 700 script.sh
: Grants full permissions to the owner and no permissions to others.
chmod 755 script.sh
ls -l script.sh
Output:
-rwxr-xr-x 1 user group 44 Nov 10 10:30 script.sh
Granting Execute Permission to Specific Users
If you want only the owner to have execute permissions:
chmod u+x script.sh
If you want to remove execute permissions for others:
chmod o-x script.sh
Best Practices for Script Permissions
1. Limit Permissions
Grant only the necessary permissions. For instance, if a script doesn’t need to be run by others, avoid giving x
permissions to the group and others:
chmod 700 script.sh
2. Use Descriptive Names
Ensure your scripts have clear and descriptive names to avoid confusion when managing multiple files.
3. Secure Sensitive Scripts
For scripts containing sensitive information, restrict access to the owner:
chmod 600 sensitive_script.sh
4. Test in a Controlled Environment
Before running a script with elevated privileges, test it in a safe environment to avoid unintended consequences.
Troubleshooting Common Issues
1. “Permission Denied” Error
If you encounter a “Permission Denied” error when running a script, ensure it has the x
permission:
chmod +x script.sh
2. Incorrect Path
If the script isn’t found, provide the correct path:
./script.sh
3. Environment Path Issues
If you want to run the script without specifying the path, ensure the script is in a directory included in the $PATH
variable:
echo $PATH
sudo mv script.sh /usr/local/bin/
Automating chmod
for Multiple Files
If you have multiple scripts to make executable, use a loop:
for file in *.sh; do
chmod +x "$file"
done
This command adds execute permissions to all .sh
files in the current directory.
Conclusion
Making a script executable in Linux using the chmod
command is a fundamental skill for anyone working with shell scripts. By understanding file permissions and how to modify them, you can effectively manage and secure your scripts. This guide covered the basics of chmod
, advanced usage, best practices, and troubleshooting tips to ensure you have a comprehensive understanding of the process.
With these insights, you can confidently create, manage, and execute scripts in your Linux environment, optimizing your workflow and enhancing your system’s capabilities.
Subscribe
Login
0 Comments
Oldest