Automate Workflows on Your Linux Server: A Beginner’s Guide to Bash Scripting
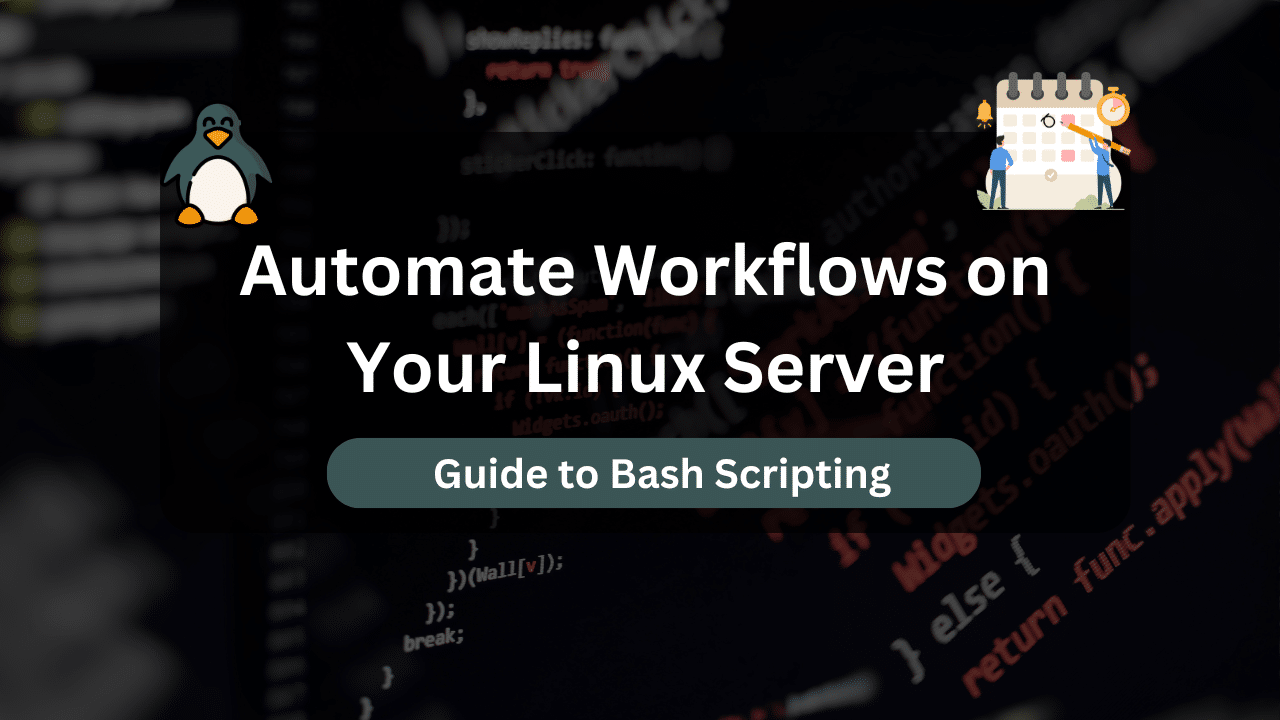
The world of server management can be a whirlwind of repetitive tasks. Manually executing these tasks wastes valuable time that could be spent focusing on more strategic initiatives. Here’s where the power of Bash scripting on Linux servers comes in. By learning even the basic concepts of Bash scripting, you can automate these repetitive tasks, streamline your workflow, and become a more efficient server administrator.
This guide is designed specifically for beginners, offering a clear and concise introduction to Bash scripting on Linux servers. We’ll delve into scripting fundamentals, explore practical examples, and equip you with the knowledge to automate common server management tasks.
Table of Contents
- Why Automate Tasks with Bash Scripting?
- Getting Started with Bash Scripting
- Building Blocks of Bash Scripting
- Practical Examples of Bash Scripting for Server Management
- Simplify Network Authentication With Our FreeRadius Servers!
- Best Practices and Tips for Effective Bash Scripting
- Beyond Server Management: Broader Applications of Bash Scripting
- Resources and Next Steps
Why Automate Tasks with Bash Scripting?
The benefits of automating tasks with Bash scripting are compelling:
- Increased Efficiency: Automating repetitive tasks frees up your time, allowing you to focus on more critical aspects of server management, such as security, performance optimization, and troubleshooting.
- Reduced Errors: Manual tasks are prone to human error. Scripts, when written correctly, execute the same commands flawlessly every time, minimizing the risk of mistakes.
- Improved Consistency: Scripts ensure tasks are performed consistently with the same parameters, guaranteeing predictable and reliable outcomes.
- Scalability: Scaling up automated tasks is as simple as copying and modifying scripts, making them ideal for managing multiple servers or complex workflows.
- Documentation and Repeatability: Scripts serve as well-documented records of the tasks they perform, simplifying future maintenance and facilitating knowledge sharing within teams.
Getting Started with Bash Scripting
- The Terminal: Your Command Center: The terminal is your gateway to interacting with your Linux server through command-line commands. Familiarize yourself with basic navigation, using commands like
ls
(list directory contents),cd
(change directory), andpwd
(show current working directory). - Learning Basic Bash Commands: Bash, the default shell on most Linux distributions, interprets and executes your commands. Common commands you’ll encounter include:
echo
: Prints text to the terminal.mkdir
: Creates directories.touch
: Creates empty files.rm
: Removes files or directories (use with caution!).cp
: Copies files.mv
: Moves or renames files.cat
: Displays the contents of a file.grep
: Searches for patterns within text files.
- Creating Your First Script: Scripts are simply text files containing a series of commands you want to execute sequentially. A common naming convention for shell scripts is using the
.sh
extension. Here’s how to create a basic script:- Open your preferred text editor (e.g.,
nano
orvim
). - Write your commands, one per line.
- Save the file with a descriptive name ending in
.sh
(e.g.,update_packages.sh
).
- Open your preferred text editor (e.g.,
- Making Your Script Executable: By default, scripts aren’t executable. To grant execution permission, use the
chmod
command:Bashchmod +x your_script.sh
- Running Your Script: Navigate to the directory containing your script and execute it using the following syntax:Bash
./your_script.sh
Building Blocks of Bash Scripting
Now that you’ve created your first script, let’s explore some fundamental concepts that will empower you to write more complex scripts:
- Variables: Variables act as named containers that store data within your script. You can assign values to variables using the syntax
variable_name=value
. - Comments: Comments are lines within your script that are ignored by the Bash interpreter. They are invaluable for explaining the purpose of specific code sections and enhancing script readability for future reference. Use
#
to add comments. - Conditional Statements: These statements allow your script to make decisions based on certain conditions. Common conditional statements include
if
,else
, andelif
. For example:Bashif [ condition ]; then # commands to execute if condition is true else # commands to execute if condition is false fi
- Loops: Loops allow you to execute a block of code repeatedly until a specific condition is met. Common loop structures include
for
loops (iterate over a sequence) andwhile
loops (execute code as long as a condition is true).
Practical Examples of Bash Scripting for Server Management
Here are some real-world examples of how Bash scripting can streamline common server management tasks:
- Automated Backups: Regular backups are crucial for data security. You can write a script to automatically backup your critical server files to an external storage location. Here’s a basic example script for automated backups:
#!/bin/bash
# Define backup directory (modify as needed)
BACKUP_DIR=/home/server/backups
# Get current date
DATE=$(date +"%Y-%m-%d")
# Create backup directory if it doesn't exist
mkdir -p $BACKUP_DIR
# Archive important files using tar
tar -cvzf $BACKUP_DIR/$DATE.tar.gz /etc /var/www
echo "Backup completed successfully to $BACKUP_DIR/$DATE.tar.gz"
This script creates a daily backup archive with the current date in the filename. Remember to adjust the script based on your specific backup requirements and directory structure.
- System Update Automation:
Keeping your server software up-to-date is essential for security and performance. A script can automate the update process:
#!/bin/bash
# Update package lists
sudo apt update
# Upgrade installed packages
sudo apt upgrade -y
# Reboot the server if required (optional)
# sudo reboot
This script updates package lists and upgrades installed packages. The -y
flag with apt upgrade
assumes a “yes” response to any prompts, so use it with caution in production environments. The commented reboot
line is optional and can be included if your system typically requires a reboot after updates.
- Disk Usage Monitoring:
Monitoring disk usage is essential for preventing storage exhaustion. A script can check disk usage and send alerts if thresholds are exceeded:
#!/bin/bash
# Define disk mount point and threshold (modify as needed)
DISK_MOUNT=/var/www
THRESHOLD=80
# Get disk usage percentage
DISK_USAGE=$(df -hP $DISK_MOUNT | awk '/[0-9]+%/ {print $5}')
# Check if usage exceeds threshold
if [[ $DISK_USAGE -ge $THRESHOLD ]]; then
echo "Disk usage on $DISK_MOUNT is critically high at $DISK_USAGE%"
# Add logic to send email alert or notification here (e.g., mail)
fi
This script checks the disk usage of a specific mount point and sends an alert (implementation not shown) if it exceeds a predefined threshold.
- Log Rotation Management:
Server logs can accumulate significant storage space. A script can automate log rotation, keeping current logs manageable:
#!/bin/bash
# Define log file path and number of backups (modify as needed)
LOG_FILE=/var/log/apache2/access.log
NUM_BACKUPS=5
# Rotate logs using logrotate utility
sudo logrotate -f /etc/logrotate.d/apache2
This script utilizes the logrotate
utility to manage log rotation for a specific log file, keeping a defined number of backups.
Simplify Network Authentication With Our FreeRadius Servers!
Experience seamless authentication management with DraculaServers’ FreeRadius Servers. Optimized for reliability and scalability, our servers ensure lightning-fast responses and robust security tailored to your network’s needs. Say goodbye to authentication headaches and streamline your network management effortlessly with DraculaServers. Learn more at Here.
Ready to simplify your network authentication? Discover the power of our FreeRadius Servers Here!
Best Practices and Tips for Effective Bash Scripting
As you delve deeper into the world of Bash scripting, here are some best practices and tips to guide you:
- Start Simple: Begin with automating basic tasks you perform regularly. This builds confidence and allows you to grasp fundamental concepts before tackling more complex scripts.
- Readability and Maintainability: Write clear and concise scripts. Use comments to explain the purpose of different code sections, making them easier to understand for yourself and others who might need to modify them in the future.
- Modularization: Break down complex tasks into smaller, reusable functions. This enhances code organization, promotes reusability, and simplifies debugging.
- Error Handling: Implement robust error handling mechanisms within your scripts. Use conditional statements to check for potential errors and include appropriate actions, such as displaying error messages or terminating the script gracefully.
- Input Validation: If your script accepts user input, validate it to ensure it meets your script’s requirements. This helps prevent unexpected behavior or security vulnerabilities.
- Testing: Thoroughly test your scripts before deploying them in a production environment. Use test data to simulate real-world scenarios and identify any potential issues.
- Security Considerations: Be mindful of security implications, especially when dealing with sensitive data or elevated privileges. Minimize the permissions granted to your scripts and avoid storing sensitive information directly within them.
- Version Control: Utilize version control systems like Git to track changes, manage script revisions, and collaborate effectively with others.
- Seek Help and Share Knowledge: Don’t be afraid to seek help online or within the Linux community forums when you encounter challenges. There’s a wealth of knowledge and support available. As you gain experience, consider sharing your scripts and knowledge to contribute to the broader community.
By following these best practices and tips, you can develop effective and secure Bash scripts that streamline your workflows and enhance your Linux server management experience.
Beyond Server Management: Broader Applications of Bash Scripting
While server management is a primary use case, Bash scripting offers a vast array of applications beyond system administration:
- Data Analysis: Automate data processing tasks, manipulate datasets, and generate reports.
- Web Scraping: Extract specific data from websites for further analysis.
- File Management: Automate repetitive file organization, renaming, or deletion tasks.
- Personal Automation: Schedule downloads, manage notifications, or streamline your digital workflow.
Resources and Next Steps
This guide has provided a foundation for understanding Bash scripting fundamentals and its value in automating server management tasks. As you embark on your scripting journey, here are some resources to empower your growth:
- Online Tutorials: Numerous online tutorials and courses cater to beginners, offering step-by-step guides and interactive exercises.
- Documentation: The Bash Guide (https://www.gnu.org/software/bash/manual/) is an excellent resource for in-depth reference and exploration of advanced scripting concepts.
- Practice and Experimentation: The best way to solidify your learning is through hands-on practice. Experiment with different scripts, explore online examples, and don’t be afraid to break things and learn from your mistakes.
By leveraging the power of Bash scripting, you can transform your server management approach, automate repetitive tasks, and free up valuable time to focus on more strategic initiatives. Remember, the key lies in starting small, practicing consistently, and exploring the vast potential of this versatile scripting language.
Check out More Linux Tutorials Here!
Subscribe
Login
0 Comments
Oldest