Bash set -e | Explained
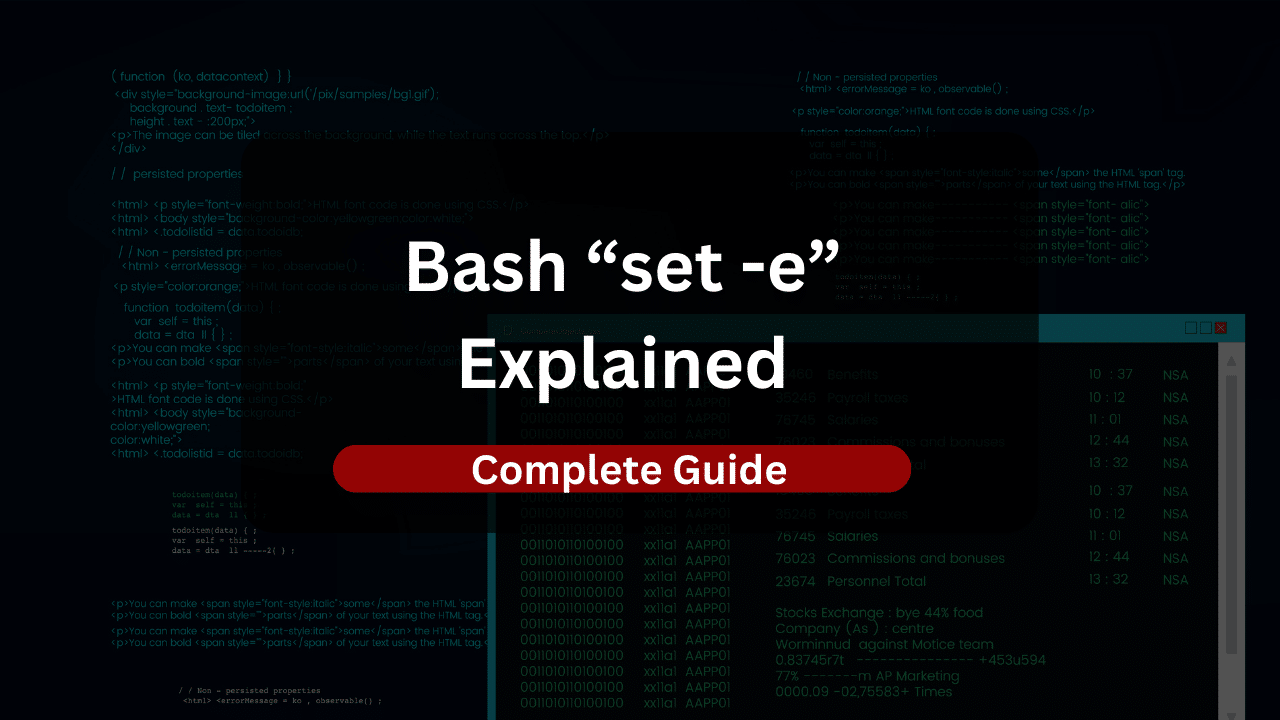
Bash scripting is a powerful tool for automating tasks, and the set -e
option plays a crucial role in enhancing the robustness of scripts. This guide will provide a comprehensive explanation of set -e
, covering its syntax, working mechanism, and practical examples.
Introduction to set -e
in Bash
In Bash scripting, set -e
is an option that instructs the script to exit immediately if any command it executes returns a non-zero status. This early exit mechanism is invaluable for improving script reliability.
Importance of set -e
The primary significance of set -e
lies in its ability to enhance script reliability. By enforcing an immediate exit upon encountering errors, it helps prevent unintended consequences and ensures that scripts halt execution if critical commands fail.
Impact on Script Execution
When set -e
is in effect, the script will cease execution if any command within it fails. This provides an early indication of issues, preventing further actions that might compound errors.
Understanding the Syntax
To activate the set -e
option in a Bash script, include the following line at the beginning of the script:
#!/bin/bash
set -e
This ensures that the script will exit if any subsequent command fails.
Working Mechanism of set -e
Let’s have a look at the working mechanism of the “set -e” in Bash scripting.
Exit on Non-Zero Status
The primary functionality of set -e
is to make the script exit if any command it runs returns a non-zero exit status. This is particularly useful for catching errors early in the script execution.
Exceptions with ||
Operator
While set -e
provides a robust way to handle errors, there are scenarios where you might want to continue execution despite a command failing. In such cases, the ||
operator can be used to handle specific commands differently.
#!/bin/bash
set -e
# Command that can fail without causing the script to exit
some_command || true
# Following commands will still trigger an exit on failure
another_command
yet_another_command
Practical Examples of “set -e” in Bash
To better understand the working and usage of the “set -e” in Bash, let’s have a look at some practical examples.
Basic Example
Let’s consider a simple script without set -e
:
#!/bin/bash
echo "Hello, World!"
# Simulate a failure
ls /nonexistent_directory
echo "Script continues..."
In this scenario, the script would continue executing even after the ls
command fails. Now, let’s add set -e
to the script:
#!/bin/bash
set -e
echo "Hello, World!"
# Simulate a failure
ls /nonexistent_directory
echo "Script continues..."
With set -e
, the script would exit immediately after the failed ls
command, and “Script continues…” would not be printed.
Advanced Example with Functions
Consider a script that includes functions. Without set -e
, the script might proceed with subsequent commands even if a function fails:
#!/bin/bash
function critical_function() {
echo "Executing critical function..."
false # Simulate failure
}
echo "Hello, World!"
# Call the critical function
critical_function
echo "Script continues..."
In this case, “Script continues…” would be printed despite the failure in the critical_function
. Now, let’s add set -e
:
#!/bin/bash
set -e
function critical_function() {
echo "Executing critical function..."
false # Simulate failure
}
echo "Hello, World!"
# Call the critical function
critical_function
echo "Script continues..."
With set -e
, the script would exit immediately after the failure in critical_function
.
Dracula VPS Hosting Service
Dracula Servers offers high-performance server hosting at entry-level prices. The plans include Linux VPS, Sneaker Servers, Dedicated Servers & turnkey solutions. If you’re looking for quality self-managed servers with high amounts of RAM and storage, look no further.
Check the plans for yourself by clicking Here!
More Examples of Bash “set -e” to Practice
Let’s dive into practical examples to illustrate the application of set -e
:
Basic Usage of set -e
Consider the following simple script (basic_example.sh
):
#!/bin/bash
set -e
echo "Executing command 1"
ls non_existent_directory
echo "Command 1 executed successfully"
echo "Executing command 2"
echo "Command 2 executed successfully"
In this example, the script attempts to list the contents of a non-existent directory. The second command should not execute due to the set -e
option. When you run the script:
$ bash basic_example.sh
The output will be:
Executing command 1
ls: cannot access 'non_existent_directory': No such file or directory
The script terminates after the first command due to the encountered error.
Handling Specific Commands
Consider a scenario where you want to continue the script even if a specific command fails. In this script (specific_command.sh
), the || true
construct is used to prevent the script from exiting on that particular error:
#!/bin/bash
set -e
echo "Executing command 1"
ls non_existent_directory || true
echo "Command 1 executed successfully"
echo "Executing command 2"
echo "Command 2 executed successfully"
When you run the script:
$ bash specific_command.sh
The output will be:
Executing command 1
ls: cannot access 'non_existent_directory': No such file or directory
Command 1 executed successfully
Executing command 2
Command 2 executed successfully
The script continues to execute after the failed command.
Combining with Conditional Statements
In this script (conditional_example.sh
), set -e
is combined with conditional statements:
#!/bin/bash
set -e
echo "Executing command 1"
ls non_existent_directory
echo "Command 1 executed successfully"
if [ -e "existing_file.txt" ]; then
echo "Executing command 2"
cat existing_file.txt
echo "Command 2 executed successfully"
fi
When you run the script:
$ bash conditional_example.sh
The output will be:
Executing command 1
ls: cannot access 'non_existent_directory': No such file or directory
The script terminates after the first command due to the encountered error. Feel free to modify these examples to suit your specific use cases and explore the behavior of set -e
in different scenarios.
Conclusion
In conclusion, set -e
is a valuable tool for Bash scriptwriters, offering a mechanism to enhance script reliability by ensuring an immediate exit on encountering errors. Understanding its syntax, working mechanism, and practical implementation through examples equips scriptwriters with a powerful tool to create more robust and error-resistant Bash scripts.
Subscribe
Login
0 Comments
Oldest