Loops in Bash Scripting with Examples
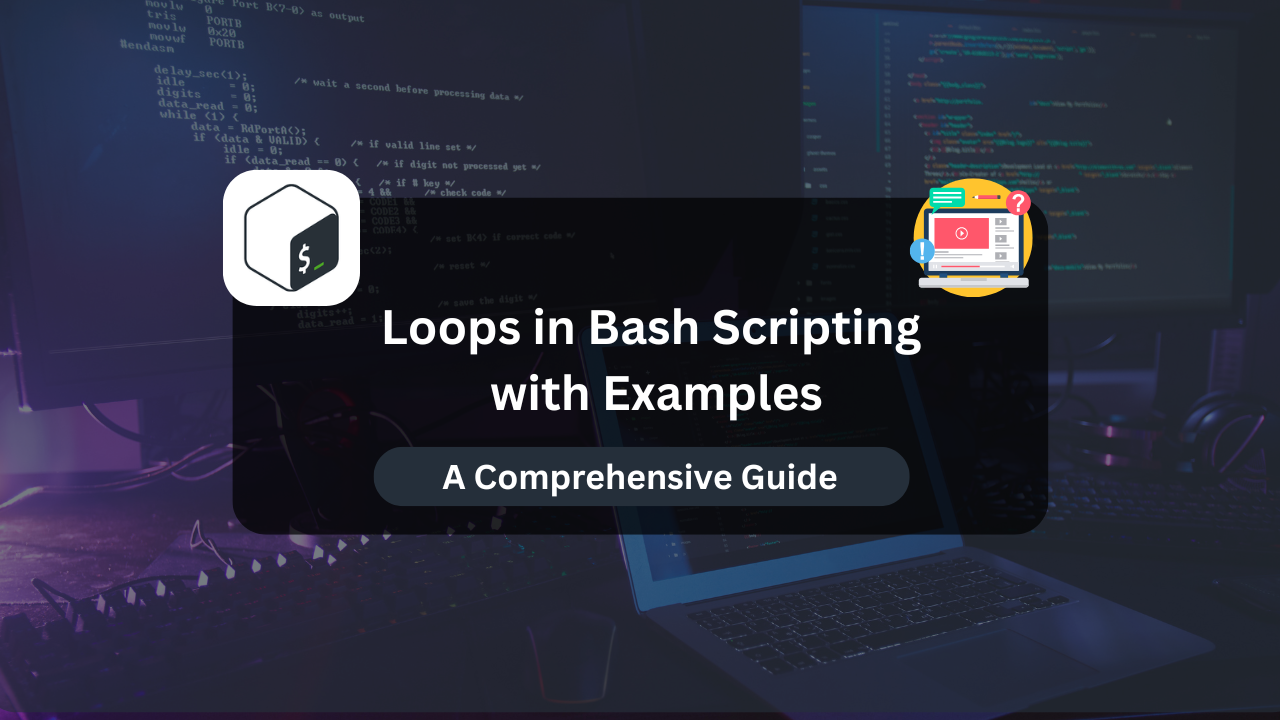
Loops are a fundamental concept in programming that allows you to execute a block of code multiple times. In Bash scripting, loops are particularly powerful for automating repetitive tasks, managing files, processing data, and more. Understanding how to use loops effectively in Bash can significantly enhance your scripting abilities.
This article provides an in-depth exploration of loops in Bash scripting, complete with examples and best practices. By the end, you’ll have a solid understanding of the various types of loops available in Bash and how to use them to streamline your tasks.
Why Use Loops in Bash Scripting?
Loops save time and effort by automating repetitive tasks. For example:
- Iterating over a list of files in a directory.
- Processing data line by line in a file.
- Running a specific command a set number of times.
Bash offers several types of loops, each suited to different scenarios. These include:
for
loopswhile
loopsuntil
loops- Loop control statements (
break
andcontinue
)
Prerequisites
Before diving into loops, ensure you have the following:
- A Linux system with Bash installed (most Linux distributions come with Bash by default).
- Basic knowledge of Bash scripting (e.g., variables, conditional statements).
- A text editor like
nano
,vim
, orgedit
to create and edit scripts.
Types of Loops in Bash Scripting
1. for
Loops
A for
loop is used to iterate over a series of items. It’s ideal for processing lists, arrays, or ranges.
Syntax:
for variable in list
do
commands
done
Example 1: Iterating Over a List
#!/bin/bash
for fruit in apple banana cherry
do
echo "I like $fruit"
done
Output:
I like apple
I like banana
I like cherry
Example 2: Iterating Over Files in a Directory
#!/bin/bash
for file in /path/to/directory/*
do
echo "Processing $file"
done
Example 3: Using a Range
#!/bin/bash
for number in {1..5}
do
echo "Number: $number"
done
Output:
Number: 1
Number: 2
Number: 3
Number: 4
Number: 5
2. while
Loops
A while
loop executes as long as a specified condition is true. It’s often used when the number of iterations is unknown.
Syntax:
while [ condition ]
do
commands
done
Example 1: Counting Down
#!/bin/bash
count=5
while [ $count -gt 0 ]
do
echo "Countdown: $count"
count=$((count - 1))
done
Output:
Countdown: 5
Countdown: 4
Countdown: 3
Countdown: 2
Countdown: 1
Example 2: Reading a File Line by Line
#!/bin/bash
while IFS= read -r line
do
echo "Line: $line"
done < input.txt
3. until
Loops
An until
loop is similar to a while
loop but executes until a specified condition becomes true.
Syntax:
until [ condition ]
do
commands
done
Example: Incrementing a Counter
#!/bin/bash
count=1
until [ $count -gt 5 ]
do
echo "Count: $count"
count=$((count + 1))
done
Output:
Count: 1
Count: 2
Count: 3
Count: 4
Count: 5
4. Nested Loops
You can nest loops within each other to handle more complex scenarios.
Example: Multiplication Table
#!/bin/bash
for i in {1..5}
do
for j in {1..5}
do
echo -n "$((i * j)) "
done
echo
done
Output:
1 2 3 4 5
2 4 6 8 10
3 6 9 12 15
4 8 12 16 20
5 10 15 20 25
5. Loop Control Statements
a. break
The break
statement exits a loop prematurely when a condition is met.
#!/bin/bash
for i in {1..10}
do
if [ $i -eq 5 ]
then
echo "Breaking the loop at $i"
break
fi
echo "Number: $i"
done
Output:
Number: 1
Number: 2
Number: 3
Number: 4
Breaking the loop at 5
b. continue
The continue
statement skips the current iteration and moves to the next.
#!/bin/bash
for i in {1..5}
do
if [ $i -eq 3 ]
then
echo "Skipping $i"
continue
fi
echo "Number: $i"
done
Output:
Number: 1
Number: 2
Skipping 3
Number: 4
Number: 5
Practical Applications of Loops in Bash
1. Batch Renaming Files
#!/bin/bash
for file in *.txt
do
mv "$file" "${file%.txt}.bak"
done
2. Monitoring System Resources
#!/bin/bash
while true
do
echo "CPU Usage: $(top -bn1 | grep "Cpu(s)" | awk '{print $2}')%"
sleep 5
done
3. Backup Script
#!/bin/bash
for file in /source/directory/*
do
cp "$file" /backup/directory/
done
Best Practices for Using Loops in Bash
- Avoid Infinite Loops: Ensure your loop conditions eventually terminate.
while : # Infinite loop do echo "Press Ctrl+C to exit" sleep 1 done
- Use Meaningful Variable Names: Choose descriptive names for loop variables.
for filename in *.jpg do echo "Processing $filename" done
- Test Loops Carefully: Test with small datasets before applying to large or critical tasks.
- Optimize for Performance: Minimize resource usage within loops, especially for large datasets.
Debugging Loops
Use the set -x
command to enable debugging and see how each command is executed:
#!/bin/bash
set -x
for i in {1..3}
do
echo "Iteration $i"
done
set +x
Conclusion
Loops are an essential tool in Bash scripting that can significantly enhance your productivity by automating repetitive tasks. This guide covered the main types of loops (for
, while
, until
), nested loops, and control statements like break
and continue
. We also explored practical applications, best practices, and debugging techniques to make the most out of loops.
By mastering loops in Bash scripting, you can write more efficient and robust scripts, streamline your workflows, and tackle complex automation challenges with confidence.
Subscribe
Login
0 Comments
Oldest